This WordPress document was very helpful in moving things around on my site, although I still have a lot of residual tweaking to do. Basically, my website that I've had for the last three years or so had the WordPress logic parked on the main page, and I wanted to move it, to make a blank, bare-bones HTML start page, to link either to the blog or to other areas of the site. The webcomic, alas, is no longer the end-all be-all of stuff I've been working on, so I wanted to marginalize it a little and focus on other things.
The base site is still the same: dscomic.com
The wordpress site will now be: dscomic.com/wordpress and will be linked from the main page.
I'm seeing a lot of strange things with images and hyperlinks, so I've still got some work to do, but so far I'm happy with the changes.
Coding Adventures
Thursday, September 22, 2011
Wednesday, June 22, 2011
Adobe Flash Professional - First test
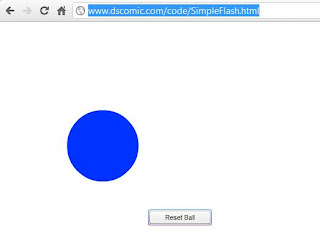
Adobe offers a decent selection of basic-level tutorials like this one, and I was able to put a simple test together and upload it to my site. Nothing fancy - just a simple animation - but an entertaining first lab and a good overview from Adobe of what some of the features are of this software. Of course, there's a mountain of complexity to this, so this is only a quick test. And only a month to go so I need to make the most of this demo... :)
Thursday, March 24, 2011
Starting with Visual C#
I've been digging into a decent book on Microsoft Visual C# 2008 by Patrice Pelland, and I really like the format of the book, as it's very hobby-programmer friendly. Not too many scary concepts or heavy coding, but rather a very visually-oriented spin through the basics of working with this particular IDE.
I'd like to check out some other titles in this series, as I think they have a good thing here for those who are still getting their feet wet in the coding world. And again the inclusion of a lot of visual images, to make sure that you are seeing what you are supposed to be seeing, is a nice touch.
Here are some of the first few programs that I put together, posted here mostly as a reference for me to revisit if needed:
Console application example:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace MyFirstConsoleApplication
{
class Program
{
static void Main(string[] args)
{
// declaring two ingeger variables that will hold
// the two parts of the sum and one integer variable to
// hold the sum;
int number1;
int number2;
int sum;
// Assigning two values to the integer variables
number1 = 10;
number2 = 5;
// adding the two integers and storing the result in the
// sum variable
sum = (number1 + number2);
// displaying a string with the two numbers and the result.
Console.WriteLine("The sum of " + number1.ToString() + " and " + number2.ToString() + " is " + sum.ToString());
}
}
}
Window Application example:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace MyFirstWindowApplication
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
// declaring two ingeger variables that will hold
// the two parts of the sum and one integer variable to
// hold the sum;
int number1;
int number2;
int sum;
// Assigning two values to the integer variables
number1 = 10;
number2 = 5;
// adding the two integers and storing the result in the
// sum variable
sum = (number1 + number2);
// displaying a string with the two numbers and the result.
MessageBox.Show("The sum of " + number1.ToString() + " and " + number2.ToString()
+ " is " + sum.ToString() + ".");
}
}
}
I'd like to check out some other titles in this series, as I think they have a good thing here for those who are still getting their feet wet in the coding world. And again the inclusion of a lot of visual images, to make sure that you are seeing what you are supposed to be seeing, is a nice touch.
Here are some of the first few programs that I put together, posted here mostly as a reference for me to revisit if needed:
Console application example:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace MyFirstConsoleApplication
{
class Program
{
static void Main(string[] args)
{
// declaring two ingeger variables that will hold
// the two parts of the sum and one integer variable to
// hold the sum;
int number1;
int number2;
int sum;
// Assigning two values to the integer variables
number1 = 10;
number2 = 5;
// adding the two integers and storing the result in the
// sum variable
sum = (number1 + number2);
// displaying a string with the two numbers and the result.
Console.WriteLine("The sum of " + number1.ToString() + " and " + number2.ToString() + " is " + sum.ToString());
}
}
}
Window Application example:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace MyFirstWindowApplication
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
// declaring two ingeger variables that will hold
// the two parts of the sum and one integer variable to
// hold the sum;
int number1;
int number2;
int sum;
// Assigning two values to the integer variables
number1 = 10;
number2 = 5;
// adding the two integers and storing the result in the
// sum variable
sum = (number1 + number2);
// displaying a string with the two numbers and the result.
MessageBox.Show("The sum of " + number1.ToString() + " and " + number2.ToString()
+ " is " + sum.ToString() + ".");
}
}
}
Tuesday, February 8, 2011
C++: Game of War!
Wrote this ages ago. Not sure if it works, but it took me forever. I'm going to periodically post some of this as I go through some of my old archives of coding school-work:
/* Rob Marsh Software September 1997 re-write #348,898
The program follows a generic path. Within the main() division it
follows the usual path:
init()
process()
closing()
But it also includes several other smaller calls to other functions,
such as the card() division, used for generation of the cards,
score(), war() {for when the players reach a draw} as well as
other functions adding definition to the program.
Most of the main action occurs within the process() division. And
it is through the closing() section that the porgram actually terminates
out of the main() division, and finishes the application. */
#include <stdio.h>
#include <stdlib.h>
/* Variable Definitions */
int comp_score; /* the computers accumulated score.*/
int ichart;
int icard;
int human_score; /* the players score throughout the game*/
int rounds = 1001;
int newchar;
int human_draw;
int comp_draw;
char win1;
int war_round = 0;
/* Below are shapes that I will use to "draw" the cards */
#define border1 201;
#define border2 205;
#define border3 187;
#define border4 186;
#define border5 200;
#define border6 188; /* these top numbers are the details the card */
#define inbord1 218;
#define inbord2 196;
#define inbord3 191;
#define inbord4 179;
#define inbord5 192;
#define inbord6 217; /* these are the interior shapes of the card */
/* Procedure definitions */
void initialization(void);
void procedure(void);
void closing(void);
void suit(void);
int card(int);
int score(int);
void war(void);
void aboutme(void);
void instructions(void);
void quit(void);
void exit(void);
void main(void)
{
/* These 3 are the main 3 sections of the program.*/
initialization();
procedure();
closing();
/* These are the other procedures to be used by the program.*/
card();
suit();
score();
war();
instructions();
aboutme();
quit();
exit();
}
void initialization (void)
{
/* Initialization will be the start menu, but it will also be
looped back to by some other areas of the program. Some menu
options will retunr here again, for another run through. */
printf("\n\n\n\n\n\n\n\n\n");
printf("\tWelcome to the game of WAR! version 1.0");
printf("\n\n\tI was designed by Rob Marsh during the fall of 1997.");
printf("\n\n\tPlease select an option:");
printf("\t\n1) Play War.");
printf("\t\n2) Read the game rules.");
printf("\t\n3) About Rob Marsh Software.");
printf("\t\n4) Quit to DOS.");
printf("\n\n\n\n\n\n\n");
ichart = \0;
scanf("%d", &ichart);
if (ichart == 2)
{
instructions();
}
else if (ichart == 3)
{
aboutme();
}
else if (ichart == 1)
{
printf("\n\n\n\n\n\n\n\nLet's play, shall we?");
quit();
}
else
{
exit();
}
}
void procedure(void)
{
/* This is the main area of the game play. Note that it is
punctuated with calls to the quit() function. I added alot of
these to give the player the option of quitting at anytime,
since the game of WAR can get unbearably dull after a very short
amount of time...very similiar to rosie ODonnel.
Random numbers are generated for the values of the cards, but
regarding score, the actual COUNT of the cards is what will
ultimately be tallied in the final score.
*/
int counter;
int hands;
printf("\n\n\n\n\nHow many hands would you like to play?");
scanf("%d", &hands);
int itracker = 1;
itracker++;
while (itracker < hands)
{
printf("\n\n\n\nI drew a...");
int card( human_draw );
printf("\nYou drew a...");
int card( comp_draw);
/* The two statements above produce the effect of 2 random
cards appearing on the screen. The commands below will
analyze which players card wins the round, or if this get's
carried over into the WAR function. */
if ( comp_draw > human_draw )
{
printf("\nI win, human!!!");
int score(comp_score);
comp_draw = (\0);
quit();
}
else if ( irandom > irandom2)
{
printf("\nYou win, human!!!");
int score(human_score);
human_draw = (\0);
quit();
}
else
{
war();
}
}
quit();
}
void closing (void)
{
printf("\n\n\n\n\nThe final score.....");
if (human_score > comp_score)
{
printf("\n\nYou win, human!");
}
else if (human_score < comp_score)
{
printf("\n\nI win, human!");
}
else
{
printf("\n\nWe tied, human!");
}
printf("\n\nThe final score: Me\t%d\tYou%d", human_score, comp_score);
printf("\nThank you for playing!");
/* this will be the final tally for the game, displayed on the screen.
of course, this is skipped if the player complied with the
quit() option and decides to jump out of the game.
Sheena Easton had a great singing voice. Where the heck
did she vanish to? */
}
int score (int x)
{
return (x + 2);
}
int card( int y )
{
int irandom = random(15);
icard = irandom;
y = icard
/* the computer will draw the design of the cards below.
while (1)
{
if (icard == 11) /* this prints up a jack card
I proceeded with possibly some unnecessary
repetition in this area, but this is due to the
fact that I had difficulty with initial printing
of the cards during some earlier versions of the
program. I couldn't quite pinpoint the problem,
so I redesigned the way ther cards were assembled,
and there is a greater focus on details. */
{
printf("%c%c%c%c%c",border1,border2,border2,border2, border3);
printf("\n");
printf("%c%J %c",border4, border4);
/* thank goodness for cut and paste */
printf("%c%c%c%c%c",border4, inbord1,inbord2,inbord3,border4);
printf("%c%c%c%c%c",border4, inbord4,"$",inbord4,border4);
printf("%c%c%c%c%c",border4, inbord5,inbord2,inbord6,border4);
printf("%c% J%c",border4, border4);
printf("%c%c%c%c%c",border5,border2,border2,border2,border6);
suit();
return (y);
break;
}
else if ( icard == 12) /* This is the Queen card */
{
printf("%c%c%c%c%c",border1,border2,border2,border2, border3);
printf("\n");
printf("%c%Q %c",border4, border4);
/* thank goodness for cut and paste */
printf("%c%c%c%c%c",border4,inbord1,inbord2,inbord3,border4);
printf("%c%c%c%c%c",border4,inbord4,"@",inbord4,border4);
printf("%c%c%c%c%c",border4,inbord5,inbord2,inbord6,border4);
printf("%c% Q%c",border4,border4);
printf("%c%c%c%c%c",border5,border2,border2,border2,border6);
suit();
return (y);
break;
}
else if (icard == 13) /* This prints up the King card...
Once again, thank goodness for cut n' paste */
{
printf("%c%c%c%c%c",border1,border2,border2,border2, border3);
printf("\n");
printf("%c%K %c",border4, border4);
/* thank goodness for cut and paste */
printf("%c%c%c%c%c",border4,inbord1,inbord2,inbord3,border4);
printf("%c%c%c%c%c",border4,inbord4,"!",inbord4,border4);
printf("%c%c%c%c%c",border4,inbord5,inbord2,inbord6,border4);
printf("%c% K%c",border4, border4);
printf("%c%c%c%c%c",border5,border2,border2,border2,border6);
suit();
return (y);
break;
}
else if (icard == 14) /* This prints up the ACE card */
{
printf("%c%c%c%c%c",border1,border2,border2,border2, border3);
printf("\n");
printf("%cACE%c",border4, border4);
/* thank goodness for cut and paste */
printf("%c%c%c%c%c",border4,inbord1,inbord2,inbord3,border4);
printf("%c%c%c%c%c",border4,inbord4,"+",inbord4,border4);
printf("%c%c%c%c%c",border4,inbord5,inbord2,inbord6,border4);
printf("%c%ACE%c",border4, border4);
printf("%c%c%c%c%c",border5,border2,border2,border2,border6);
suit();
return (y);
break;
}
else if ((icard > 1) && (icard < 11))
{
printf("%c%c%c%c%c",border1,border2,border2,border2, border3);
printf("\n");
printf("%c%3d%c",border4, icard, border4);
/* thank goodness for cut and paste */
printf("%c%c%c%c%c",border4,inbord1,inbord2,inbord3,border4);
printf("%c%c%c%c%c",border4,inbord4,"*",inbord4,border4);
printf("%c%c%c%c%c",border4,inbord5,inbord2,inbord6,border4);
printf("%c%3d%c",border4,icard, border4);
printf("%c%c%c%c%c",border5,border2,border2,border2,border6);
suit();
return (y);
break;
}
else
{
/* The condition met was a one or a zero, and ....
"I can't go for that! no can do!"
this condition should only be met if the random number
is a 1 or a zero. The while loop will continue without
stopping, and hopefully it will re-value the icard with a
new number that fits into one of the above categories.*/
}
}
}
void suit(void)
/* suit actually has no real bearing on the game regarding how
points are accumulated, but it helps make the cards
seem like cards. I couldn't get the symbols to print
as of the latest re-write of this program. According to the
ANSI symbol list, it should simply be 'alt-001 through 004'
but for some reason it wouldn't accept these.
I would prefer to simply have these symbols, but since
I dont, the sentences below will have to suffice. */
{
while(1)
{
int irandom3 = random(5);
if (irandom3 == 1)
{
printf("\nSuit of Hearts");
break;
}
else if (irandom3 == 2)
{
printf("\nSuit of Clubs");
break;
}
else if (irandom3 == 3)
{
printf("\nSuit of Spades");
break;
}
else if (irandom3 == 4)
{
printf("\nSuit of Diamonds");
break;
}
else
{
/* returns to loop to retrieve another value */
}
}
}
void war(void)
{
printf("\n\nWar is declared, human!");
quit();
int irounds = (\0);
while (1)
{
int irounds = irounds + 6;
printf("\nI drew 3 cards, the face card is...");
int card (comp_draw);
printf("\nYou drew 3 cards, the face card is...");
int card(human_draw);
if (comp_draw > human_draw)
{
comp_score = (comp_score + irounds);
break;
}
else if (human_draw > comp_draw)
{
human_score = (human_score + irounds);
break;
}
else
{
printf("\nWe tied, so we play again!");
quit();
}
}
}
void quit(void)
{
printf("\n\nHit any key to continue, or CTRL-C to quit");
getchar();
}
void instructions(void)
{
printf("\n\n\n\n\n\n\n\n");
printf("\nInstructions");
printf("\n\nThe game of War!");
printf("\n\nIn this game, the two players will be you");
printf("\nversus the computer. The computer has been programmed by me to have a ");
printf("\nremarkable, unrivaled artificial intelligence. He will be, quit possibly,");
printf("\nthe most brilliant opponent to ever face you in the card game of war!");
printf("\n\nIn War, 2 players draw a card from the deck. The player who draws the");
printf("\nhigher card wins both cards. If both cards contain the same face value");
printf("\nthen WAR is declared! The game then goes into a war round. Both players");
printf("\ndraw 3 cards, 1 face up and 2 face down. The player who holds the higher");
printf("\nface value of the card wins all the cards! If both cards are the same,");
printf("\nwell, then, the war round continues, the sequence continues until one of the");
printf("\nplayers has a higher card than the other. When a winner is determined, he");
printf("\ntakes ALL the cards of the war round!");
printf("\n\n");
printf("The game is easy to play, and easy to master.");
printf("Get ready to face the computer...a master of WAR!");
quit();
initialization();
}
void aboutme(void)
{
printf("\n\n\n\n\n\n\n\n");
printf("\nAbout me");
printf("\n\nRob Marsh is a struggling computer student, who is too stupid to admit");
printf("\nThat he is probably taking more classes than he can handle. ");
quit();
initialization();
}
void exit(void)
{
exit(0);
}
/* Rob Marsh Software September 1997 re-write #348,898
The program follows a generic path. Within the main() division it
follows the usual path:
init()
process()
closing()
But it also includes several other smaller calls to other functions,
such as the card() division, used for generation of the cards,
score(), war() {for when the players reach a draw} as well as
other functions adding definition to the program.
Most of the main action occurs within the process() division. And
it is through the closing() section that the porgram actually terminates
out of the main() division, and finishes the application. */
#include <stdio.h>
#include <stdlib.h>
/* Variable Definitions */
int comp_score; /* the computers accumulated score.*/
int ichart;
int icard;
int human_score; /* the players score throughout the game*/
int rounds = 1001;
int newchar;
int human_draw;
int comp_draw;
char win1;
int war_round = 0;
/* Below are shapes that I will use to "draw" the cards */
#define border1 201;
#define border2 205;
#define border3 187;
#define border4 186;
#define border5 200;
#define border6 188; /* these top numbers are the details the card */
#define inbord1 218;
#define inbord2 196;
#define inbord3 191;
#define inbord4 179;
#define inbord5 192;
#define inbord6 217; /* these are the interior shapes of the card */
/* Procedure definitions */
void initialization(void);
void procedure(void);
void closing(void);
void suit(void);
int card(int);
int score(int);
void war(void);
void aboutme(void);
void instructions(void);
void quit(void);
void exit(void);
void main(void)
{
/* These 3 are the main 3 sections of the program.*/
initialization();
procedure();
closing();
/* These are the other procedures to be used by the program.*/
card();
suit();
score();
war();
instructions();
aboutme();
quit();
exit();
}
void initialization (void)
{
/* Initialization will be the start menu, but it will also be
looped back to by some other areas of the program. Some menu
options will retunr here again, for another run through. */
printf("\n\n\n\n\n\n\n\n\n");
printf("\tWelcome to the game of WAR! version 1.0");
printf("\n\n\tI was designed by Rob Marsh during the fall of 1997.");
printf("\n\n\tPlease select an option:");
printf("\t\n1) Play War.");
printf("\t\n2) Read the game rules.");
printf("\t\n3) About Rob Marsh Software.");
printf("\t\n4) Quit to DOS.");
printf("\n\n\n\n\n\n\n");
ichart = \0;
scanf("%d", &ichart);
if (ichart == 2)
{
instructions();
}
else if (ichart == 3)
{
aboutme();
}
else if (ichart == 1)
{
printf("\n\n\n\n\n\n\n\nLet's play, shall we?");
quit();
}
else
{
exit();
}
}
void procedure(void)
{
/* This is the main area of the game play. Note that it is
punctuated with calls to the quit() function. I added alot of
these to give the player the option of quitting at anytime,
since the game of WAR can get unbearably dull after a very short
amount of time...very similiar to rosie ODonnel.
Random numbers are generated for the values of the cards, but
regarding score, the actual COUNT of the cards is what will
ultimately be tallied in the final score.
*/
int counter;
int hands;
printf("\n\n\n\n\nHow many hands would you like to play?");
scanf("%d", &hands);
int itracker = 1;
itracker++;
while (itracker < hands)
{
printf("\n\n\n\nI drew a...");
int card( human_draw );
printf("\nYou drew a...");
int card( comp_draw);
/* The two statements above produce the effect of 2 random
cards appearing on the screen. The commands below will
analyze which players card wins the round, or if this get's
carried over into the WAR function. */
if ( comp_draw > human_draw )
{
printf("\nI win, human!!!");
int score(comp_score);
comp_draw = (\0);
quit();
}
else if ( irandom > irandom2)
{
printf("\nYou win, human!!!");
int score(human_score);
human_draw = (\0);
quit();
}
else
{
war();
}
}
quit();
}
void closing (void)
{
printf("\n\n\n\n\nThe final score.....");
if (human_score > comp_score)
{
printf("\n\nYou win, human!");
}
else if (human_score < comp_score)
{
printf("\n\nI win, human!");
}
else
{
printf("\n\nWe tied, human!");
}
printf("\n\nThe final score: Me\t%d\tYou%d", human_score, comp_score);
printf("\nThank you for playing!");
/* this will be the final tally for the game, displayed on the screen.
of course, this is skipped if the player complied with the
quit() option and decides to jump out of the game.
Sheena Easton had a great singing voice. Where the heck
did she vanish to? */
}
int score (int x)
{
return (x + 2);
}
int card( int y )
{
int irandom = random(15);
icard = irandom;
y = icard
/* the computer will draw the design of the cards below.
while (1)
{
if (icard == 11) /* this prints up a jack card
I proceeded with possibly some unnecessary
repetition in this area, but this is due to the
fact that I had difficulty with initial printing
of the cards during some earlier versions of the
program. I couldn't quite pinpoint the problem,
so I redesigned the way ther cards were assembled,
and there is a greater focus on details. */
{
printf("%c%c%c%c%c",border1,border2,border2,border2, border3);
printf("\n");
printf("%c%J %c",border4, border4);
/* thank goodness for cut and paste */
printf("%c%c%c%c%c",border4, inbord1,inbord2,inbord3,border4);
printf("%c%c%c%c%c",border4, inbord4,"$",inbord4,border4);
printf("%c%c%c%c%c",border4, inbord5,inbord2,inbord6,border4);
printf("%c% J%c",border4, border4);
printf("%c%c%c%c%c",border5,border2,border2,border2,border6);
suit();
return (y);
break;
}
else if ( icard == 12) /* This is the Queen card */
{
printf("%c%c%c%c%c",border1,border2,border2,border2, border3);
printf("\n");
printf("%c%Q %c",border4, border4);
/* thank goodness for cut and paste */
printf("%c%c%c%c%c",border4,inbord1,inbord2,inbord3,border4);
printf("%c%c%c%c%c",border4,inbord4,"@",inbord4,border4);
printf("%c%c%c%c%c",border4,inbord5,inbord2,inbord6,border4);
printf("%c% Q%c",border4,border4);
printf("%c%c%c%c%c",border5,border2,border2,border2,border6);
suit();
return (y);
break;
}
else if (icard == 13) /* This prints up the King card...
Once again, thank goodness for cut n' paste */
{
printf("%c%c%c%c%c",border1,border2,border2,border2, border3);
printf("\n");
printf("%c%K %c",border4, border4);
/* thank goodness for cut and paste */
printf("%c%c%c%c%c",border4,inbord1,inbord2,inbord3,border4);
printf("%c%c%c%c%c",border4,inbord4,"!",inbord4,border4);
printf("%c%c%c%c%c",border4,inbord5,inbord2,inbord6,border4);
printf("%c% K%c",border4, border4);
printf("%c%c%c%c%c",border5,border2,border2,border2,border6);
suit();
return (y);
break;
}
else if (icard == 14) /* This prints up the ACE card */
{
printf("%c%c%c%c%c",border1,border2,border2,border2, border3);
printf("\n");
printf("%cACE%c",border4, border4);
/* thank goodness for cut and paste */
printf("%c%c%c%c%c",border4,inbord1,inbord2,inbord3,border4);
printf("%c%c%c%c%c",border4,inbord4,"+",inbord4,border4);
printf("%c%c%c%c%c",border4,inbord5,inbord2,inbord6,border4);
printf("%c%ACE%c",border4, border4);
printf("%c%c%c%c%c",border5,border2,border2,border2,border6);
suit();
return (y);
break;
}
else if ((icard > 1) && (icard < 11))
{
printf("%c%c%c%c%c",border1,border2,border2,border2, border3);
printf("\n");
printf("%c%3d%c",border4, icard, border4);
/* thank goodness for cut and paste */
printf("%c%c%c%c%c",border4,inbord1,inbord2,inbord3,border4);
printf("%c%c%c%c%c",border4,inbord4,"*",inbord4,border4);
printf("%c%c%c%c%c",border4,inbord5,inbord2,inbord6,border4);
printf("%c%3d%c",border4,icard, border4);
printf("%c%c%c%c%c",border5,border2,border2,border2,border6);
suit();
return (y);
break;
}
else
{
/* The condition met was a one or a zero, and ....
"I can't go for that! no can do!"
this condition should only be met if the random number
is a 1 or a zero. The while loop will continue without
stopping, and hopefully it will re-value the icard with a
new number that fits into one of the above categories.*/
}
}
}
void suit(void)
/* suit actually has no real bearing on the game regarding how
points are accumulated, but it helps make the cards
seem like cards. I couldn't get the symbols to print
as of the latest re-write of this program. According to the
ANSI symbol list, it should simply be 'alt-001 through 004'
but for some reason it wouldn't accept these.
I would prefer to simply have these symbols, but since
I dont, the sentences below will have to suffice. */
{
while(1)
{
int irandom3 = random(5);
if (irandom3 == 1)
{
printf("\nSuit of Hearts");
break;
}
else if (irandom3 == 2)
{
printf("\nSuit of Clubs");
break;
}
else if (irandom3 == 3)
{
printf("\nSuit of Spades");
break;
}
else if (irandom3 == 4)
{
printf("\nSuit of Diamonds");
break;
}
else
{
/* returns to loop to retrieve another value */
}
}
}
void war(void)
{
printf("\n\nWar is declared, human!");
quit();
int irounds = (\0);
while (1)
{
int irounds = irounds + 6;
printf("\nI drew 3 cards, the face card is...");
int card (comp_draw);
printf("\nYou drew 3 cards, the face card is...");
int card(human_draw);
if (comp_draw > human_draw)
{
comp_score = (comp_score + irounds);
break;
}
else if (human_draw > comp_draw)
{
human_score = (human_score + irounds);
break;
}
else
{
printf("\nWe tied, so we play again!");
quit();
}
}
}
void quit(void)
{
printf("\n\nHit any key to continue, or CTRL-C to quit");
getchar();
}
void instructions(void)
{
printf("\n\n\n\n\n\n\n\n");
printf("\nInstructions");
printf("\n\nThe game of War!");
printf("\n\nIn this game, the two players will be you");
printf("\nversus the computer. The computer has been programmed by me to have a ");
printf("\nremarkable, unrivaled artificial intelligence. He will be, quit possibly,");
printf("\nthe most brilliant opponent to ever face you in the card game of war!");
printf("\n\nIn War, 2 players draw a card from the deck. The player who draws the");
printf("\nhigher card wins both cards. If both cards contain the same face value");
printf("\nthen WAR is declared! The game then goes into a war round. Both players");
printf("\ndraw 3 cards, 1 face up and 2 face down. The player who holds the higher");
printf("\nface value of the card wins all the cards! If both cards are the same,");
printf("\nwell, then, the war round continues, the sequence continues until one of the");
printf("\nplayers has a higher card than the other. When a winner is determined, he");
printf("\ntakes ALL the cards of the war round!");
printf("\n\n");
printf("The game is easy to play, and easy to master.");
printf("Get ready to face the computer...a master of WAR!");
quit();
initialization();
}
void aboutme(void)
{
printf("\n\n\n\n\n\n\n\n");
printf("\nAbout me");
printf("\n\nRob Marsh is a struggling computer student, who is too stupid to admit");
printf("\nThat he is probably taking more classes than he can handle. ");
quit();
initialization();
}
void exit(void)
{
exit(0);
}
Tuesday, December 28, 2010
HTML and Javascript Basics
I've been reading through "HTML and Javascript Basics" by Karl Barksdale, which is an easy-to-follow intro to Javascript. Feels like a high school text, but I'm fine with that, as the examples are simple and straight-forward for dummies like me.
I've looked through a number of programming books, and I appreciate the books that include details of the code samples to incorporate. Quite a few books seem to take the shortcut of either referring you to code on the CD-ROM (or on a website) when often, I like to just prop open a book and work/code directly from that, instead of having to bounce around all over the place looking for code samples. Also, having the code in a book makes it easier to annotate comments and notes while going through it as well.
Anyhow, I've been experimenting with some of the links on the Dad's Toenail pages, including some modifications to the navbar on the side. Nothing fancy here: in fact, the whole point of this blog is just to have a repository where I can document code that I work on.
<html>
<head>
<title>Navbar</title>
</head>
<body>
<div align="center"><b>Contents</b></div>
<ul>
<li><a href="dadstoenail.html" target="RightFrame">HOME</a></li>
<li><a href="about.html" target="RightFrame">ABOUT</a></li>
<li><a href="preview.html" target="RightFrame">PREVIEW</a></li>
<li><a href="buy.html" target="RightFrame">BUY</a></li>
<li><a href="andy.html" target="RightFrame">ANDY</a></li>
<li><a href="rob.html" target="RightFrame">ROB</a></li>
<li><a href="contact.html" target="RightFrame">CONTACT</a></li>
</ul>
</body>
</html>
I've looked through a number of programming books, and I appreciate the books that include details of the code samples to incorporate. Quite a few books seem to take the shortcut of either referring you to code on the CD-ROM (or on a website) when often, I like to just prop open a book and work/code directly from that, instead of having to bounce around all over the place looking for code samples. Also, having the code in a book makes it easier to annotate comments and notes while going through it as well.
Anyhow, I've been experimenting with some of the links on the Dad's Toenail pages, including some modifications to the navbar on the side. Nothing fancy here: in fact, the whole point of this blog is just to have a repository where I can document code that I work on.
<html>
<head>
<title>Navbar</title>
</head>
<body>
<div align="center"><b>Contents</b></div>
<ul>
<li><a href="dadstoenail.html" target="RightFrame">HOME</a></li>
<li><a href="about.html" target="RightFrame">ABOUT</a></li>
<li><a href="preview.html" target="RightFrame">PREVIEW</a></li>
<li><a href="buy.html" target="RightFrame">BUY</a></li>
<li><a href="andy.html" target="RightFrame">ANDY</a></li>
<li><a href="rob.html" target="RightFrame">ROB</a></li>
<li><a href="contact.html" target="RightFrame">CONTACT</a></li>
</ul>
</body>
</html>
Wednesday, December 22, 2010
Intro
I do enough hobby coding that I want to start documenting my notes during this progress, and blogger is the perfect place to host these thoughts. More to come.
Subscribe to:
Posts (Atom)